Threads, in C, C++, Java, PHP, Python or any other programming language, are independent execution flows from the same program.
Threads are functions that run at “background”, ie, while the program performs the main()
function - in C, a thread may be running while main() in parallel.
So we can say that programming with threads is the equivalent of programming multitasking.
Example context
My students, who are starting in programming techniques using C language, are challenged with the implementation of a simple game in text, and always come across the desire / need to show the time or clock of the game in parallel withexecution of the main logic.
In this short article I present the implementation of an example that performs two loops - ties, in the main routine (main) however, which at the same time displays in a corner of the screen the time elapsed in seconds.
In addition to demonstration of C programming with Threads in C, you can verify the use of routines such as gotoxy
- Cursor positioning on screen or console, and sleep
- pause of source code execution.
About threads
We will improve the concept of introduction, a simplified explanation is to say that they are “channels” of routine execution in systems, allowing various functions to be processed in parallel in the same application.
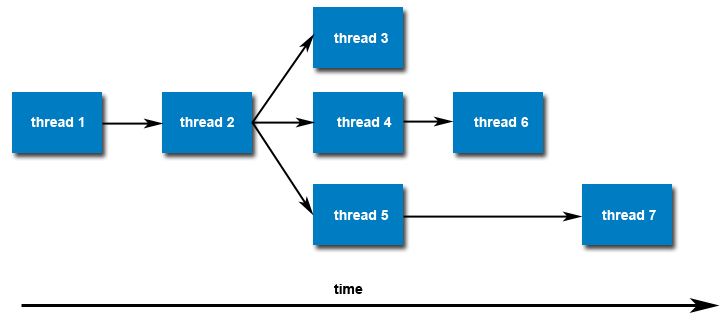
Example of programming and execution on threads running in parallel on the timeline
Thus, programming with threads is the equivalent of programming multitasking or multithreading.
Multithreading is a specialized form of multitasking, and multitasking is the feature that allows the computer to perform two or more programs or functions - routines, simultaneously.
In general, there are two types of multitasking: process -based and thread -based.
Process -based multitasking deals with simultaneous programs.The multitasking, which will be demonstrated in this article, is based on thread and deals with the simultaneous execution of parts of the same program.
A multithread program contains two or more parts that can be executed simultaneously.Each part of this program is called thread and each thread defines a separate execution path - as exemplified in the figure above.
The C programming language does not contain any embedded support for multithread applications.Instead, it depends entirely on the operating system to provide this feature.
In our simple example, the beginthread()
process library function is being used to show running time in seconds, while two repetition bonds are processed on the main thread (function).
Programming environment
For the following example the following programming environment was configured and used for code creation and execution:
Clock code using threads in programming language C
Following is the code of the example in full:
#include <stdio.h>
#include <stdlib.h>
#include <process.h>
#include <conio2.h>
int executando; // global variable that controls if the application is executing
// she had guaranteed the closing of open threads when their value is zero
void relogio () {
// The Long Type Holds Nonfractional Numbers
// with upper value (long) to type
int long inicio = time(NULL);
long agora;
while (executando != 0) {
agora = time(NULL);
// Position the watch in column 1 line 1
// The text screen has 80 columns for about 23 lines
gotoxy(1,1);
printf ("Tempo: %d\n", agora - inicio);
// This command holds the execution of the code for time in milliseconds passed by parameter
// In this case we hold in 1 second
_sleep(1000);
}
printf ("Relogio parado!\n\n");
}
int main(int argc, char *argv[]) {
int x;
executando = 1;
// starts the thread that performs the clock function in parallel with the rest of the application
_beginthread(relogio, 0, NULL);
for (x=0; x<10000; x++) {
// Position the text in column 1 line 5
// The text screen has 80 columns for about 23 lines
gotoxy(1,5);
printf ("For do MAIN %d\n", x);
}
for (x=0; x // Position the clock in column 1 line 10
// The text screen has 80 columns for about 23 lines
gotoxy(1,10);
printf ("Novo For do MAIN %d\n", x);
}
// Seating the value of this variable as 0 we guarantee that the While from the clock to the
execution
executando = 0;
system("PAUSE");
return 0;
}
Compile the code and execute it, you will see the second count while the system is performing the function main()
.
Comments