PHP Laravel is an application framework based on a Open Source Code, aimed at developing web systems with expressive and elegant syntax. It is developed in PHP, being easily adopted and learning.
Its first public version was a beta released in mid-2009.
Its web structure provides an infrastructure and starting point for creating your app, allowing you to focus on your software’s business rules.
Let framework take care of the details such as data sanitization, user input validation rules, HTTP request header treatment, HTTP response structure formatting, among many other technical features.
The Laravel Framework strives to provide an excellent experience to the developer, providing powerful features such as full dependency injection, a layer of expressive database abstraction, queues and scheduled jobs, unit and integration testing and more.
Whether you’re new to PHP or web frameworks, or have years of experience, Laravel is a framework that can keep you on growing as a developer or team.
Frameworks are known to incorporate a number of good practices and consolidated design standards to ensure robust software architecture, Laravel do this role very well.
Agora que você já sabe o que é o Framework PHP Laravel, conheça um pouco da sua estrutura e qualidade nos tópicos a seguir. Não deixe de ler também o artigo que apresenta uma visão completa dos [Componentes de arquitetura do Framework PHP Laravel].
Now that you already know what the Laravel PHP Framework is, get to know some of its elements and structure in the following topics. Do not miss reading also the article that presents a complete view of the Laravel PHP Framework Architecture Components.
Overview of the Laravel Framework
Laravel is the best choice among PHP frameworks for building modern, robust and scalable web applications.
Release Cycle
Both the Laravel framework itself, and its other original packages follow the Semantic Version Control - SEMVER.
The main versions (major) of the framework are released annually in September, while smaller versions (minor) and patch can be released weekly. Secondary and patch versions should never contain important changes that break compatibility.
[semver]: Semantic Versioning: oficial documentation at https://semver.org/
Laravel 8
By writing this article the Laravel was in its version of number 8, as main features it maintains the improvements made in Laravel 7.x by introducing Laravel Jetstream, model factory classes, squashing migrations, job batching (batch jobs), improved rate throttling, queue improvements, dynamic Blade components, paging views with Tailwind, time tests helpers, artisan serve
improvements, event listener improvements and a variety of other bug fixes and usability improvements.
For a deeper detailing of the improvements in this version, read the article [Laravel 8][2].
Know now the general characteristics of the Laravel project and Framework:
Support Policy
The Laravel project provides tools and components to increase the productivity and quality of the developed projects.
LTS (Long Term Support - Long-Term Support) versions ensure that certain versions of framework will receive bug fixes for 2 (two) years and security troubleshooting - security cools for 3 (three) years. For other versions, in general, bug fixes will be performed for 7 (seven) months and security for 1 (one) year.
For LTS versions, such as Laravel 6, bug fixes are provided for 2 years and security fixes are provided for 3 years. These versions provide the longest support and maintenance window.
For general releases, bug fixes are provided for 18 months and security fixes are provided for 2 years. For all additional libraries, including Lumen, only the latest version receives bug fixes.
Version | Release | Bug Fixes up to | Security Fixes up to |
---|---|---|---|
6 (LTS) | 7 September, 2021 | 6 September, 2022 | |
7 | March 3rd, 2020 | October 6th, 2020 | March 3rd, 2021 |
8 | September 8th, 2020 | March 1st, 2022 | September 6th, 2022 |
9 (LTS) | September, 2021 | September, 2023 | September, 2024 |
10 | September, 2022 | March, 2024 | September, 2024 |
Table of releases Laravel with maintenance time of fix Bugs and Security
Progressive structure
The main team of the Laravel project likes to call it “progressive” framework. With this, they mean that Laravel is able to track the growth of its project and, in the case of junior programmers or beginners, its professional development.
If you are just taking the first steps in web development, in addition to the vast library of documentation, guides and tutorials, it is important to know that the community around the project is very large, world-wide and with excellent professionals willing to assist beginners.
Are you a senior developer? Even better, Laravel offers robust tools for dependence injection, unit test, queues, real-time events and more. The framework is set to build professional web applications and ready to handle corporate workloads.
Scalable Structure
The PHP language when used with good software architecture concepts, offers high scalability to the systems created with it. So it’s easy to understand how Laravel can be incredibly scalable.
In addition to the friendly nature of PHP scaling, thanks to the excellent architecture employed in the Laravel framework, and the built-in support for fast and distributed cache systems like Redis, scale horizontally with Laravel is very simple. In fact, there are hundreds of commercial apps made with Laravel that were easily scaled to handle hundreds of millions of requests per month.
Need extreme scalability? Platforms such as Laravel Vapor let you run your Laravel app on an almost unlimited scale in the latest Amazon AWS serverless technology.
Community framework
Laravel organizes in its structure the best packages in the PHP language ecosystem to offer the most robust and friendly framework available to the developer. In addition, thousands of talented developers from around the world contribute to their structure. You can also become a Laravel contributor, check for example my contributions accepted to the project: PRs of @nunomazer.
Project Design Patterns in Laravel
Being a framework that applies a robust software architecture, valuing good practices of source code development and structuring, it employs several software design patterns in its infrastructure.
The following list presents the main design patterns implemented by the Laravel framework and describes an overview of each:
MVC - Model View Controller
Model-View-Controller is a software architecture pattern that separates the representation of information from the control of user interaction and business rules.
The model (model) is responsible for managing application data, business rules, logic and functions.
A view (view) is any data representation output, such as an HTML screen, report, table, or dataset in JSON or XML format. It is possible to have multiple views of the same dataset, such as a bar chart for management and a tabular view for a master data.
The controller (controller) mediates the input (customer requests), converting it into orders for the model or view. The controller is responsible for managing (orchestrating) the service flow to a request.
Facade
In software design patterns, a facade (a word of French origin) is an object that makes available a simplified interface for one of the functionalities of an API, for example.
A facade can make a software library easier to understand and use, or reduce dependencies on a library’s internals.
Service Provider
An application is an aggregation of cohesive services.
While an application offers a broad set of functionality in terms of programming interfaces (APIs) and classes, a service provides access to some specific functionality or features. The service defines interfaces to functionality and a way to retrieve an implementation.
For example, consider an application that provides a variety of information about a geographic location, such as real estate data, weather information, demographics, etc. The weather service, a part of the application, can only define the interface for retrieving weather information.
The service provider interface (SPI) is the set of public interfaces and abstract classes that a service defines. The SPI can be represented by a single interface (type) or abstract class or a set of interfaces or abstract classes that define the service contract.
IoC - Inversion of Control
Inversion of Control (IoC) is the name given to the development pattern of computer programs where the sequence (control) of method calls is inverted in relation to traditional programming, that is, it is not determined directly by the programmer.
This control is delegated to a software infrastructure often called a container or any other component that can take control over execution. This is a very common feature in some frameworks.
In Laravel, the entry point for requests is unique, through the public/index.php
file, which instantiates the main controller, or front controller, which is responsible for the inversion of control, identifying by the request parameters which method of which controller should respond to it.
DI - Dependency Injection
Dependency Injection is used to maintain a low level of coupling between different modules and components of a system.
In this standard, dependencies between components are not defined by direct instantiations by programmers, but by the configuration or standardization use of a software infrastructure (container) that is responsible for “injecting” its declared dependencies into each component.
Dependency Injection is related to the Inversion of Control pattern but cannot be considered a synonym for it.
Front Controller and Dispatcher
The front controller software design pattern defines a single entry point to your web application that handles all HTTP requests. For example in PHP: index.php
, in Laravel specifically public/index.php
This code is responsible for loading dependencies, executing security rules, internationalization, user authentication.
Used in conjunction with the Dispatcher design pattern, it uses strategies for the MVC application where the controller module (front controller) sends the processing to a dispatcher (dispatcher) which selects, based on the context of the request (parameters received by the HTTP request), the correct controller for executing the logic related to the request made.
Front Controller is a design pattern that, together with M-V-C, enables the implementation of IoC - Inversion of Control in Web systems.
Observer
Defines a one-to-many dependency between objects so that when an object changes its state, all its dependents are automatically notified and updated.
Allows interested objects (observers) to be notified of state changes or other events occurring in another object.
The Observer pattern is also called Publisher-Subscriber, Event Generator, and Dependents.
In the Laravel framework it is implemented with the concept of Listener.
ORM and ActiveRecord
ORM (Object Relational Mapping) is an approach used to persist business object (application) information in a relational database (SQLite, Oracle, Sybase, PostgreSQL, Mysql, etc).
It performs, through annotations in the classes that will represent the entities in memory, in the object-oriented pattern, the mapping with the tables and fields of the relational database.
Active record is a design pattern where the interface of a given object has actions to manipulate its data, such as Insert (Insert), Update (Update), Delete (Delete) and access properties and actions that directly correspond to the associated database columns.
An instance of an object is associated with a single record (tuple) in the table. After creating and saving an object, a new record is added to the table. A loaded object gets its information from the database of data. When an object is updated, the corresponding record in the table is also updated.
Laravel Features and Functionality
To end the presentation of the framework, we will list some of the functionalities delivered to the developer natively in its structure, providing productivity and security for your application.
Authentication
The Laravel framework provides the implementation of standardized authentication in a simple way. This feature simplifies the development of applications with little complexity and simple authentication requirements, for systems with specific requirements, such as the use of access directories (eg LDAP), OAUTH, it is possible to override the default implementation.
It even provides packages for implementing authentication via tokens for RestFul APIs.
Routing
Modeling service routes from requests to resources and actions of your system.
Routes are specified by context like web requests for classic apps, API, console .
It also offers limiting control of requests per minute and per client IP.
Security filters
The framework easily protects your application from cross-site request forgeries (CSRF) attacks; applies sanitization of data inputs and allows the implementation of middleware for other filters if needed.
Cache
Provides a unified API for various application caching systems. By specifying a driver, the framework uses it by default throughout your application.
Laravel supports tools like Memcached and Redis. By default, it is configured to use the drive file, which stores serialized objects in the file system. For large applications, it is recommended that you use an in-memory cache such as Memcached or APC.
Events
Events in Laravel provide a simple implementation of the observer pattern, allowing classes/objects to subscribe to and listen to (listeners) events in your application.
Event classes are typically stored in the app/Events
directory, while their listeners are stored in the app/Listeners
directory.
An example of use would be the creation of a UserCreated
event, which, when triggered, allows its listeners to perform actions such as sending email, creating specific profiles, validating access after creating a user.
Final considerations
If your development Stack is based on the PHP programming language, but even if you work in a company or team that does not adopt the Laravel framework, it is essential to know its potential and, at least, have an overview of its characteristics and functionalities because, with around 22 million downloads by site metrics Packagist.com, it should not be ignored.
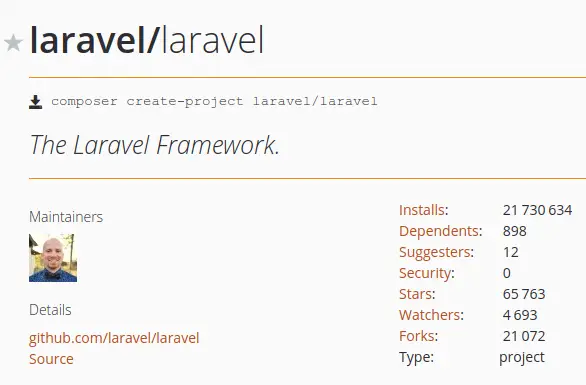
However, if you are interested in learning more about this framework, want to adopt it in your stack and want to learn how to program in Laravel, follow this blog to receive articles on good practices and problem solving in the “Laravel way”.
Click here and read articles about Laravel and Web Development.
Comments